Have you ever faced your code getting slower as you add more things to your project? This can be frustrating, especially when you’re trying to create a smooth, responsive user experience. The best part is that you can make your JavaScript code faster by using Asynchronous Techniques. In this blog post, we’ll explore how you can achieve this, even if you’re new to programming.
Table of Contents
8 Asynchronous Techniques
1. Introduction to Asynchronous JavaScript
Synchronous vs Asynchronous JavaScript
In Synchronous JavaScript, tasks are completed one after another. This means that the task must wait for the previous one to finish. For example, if you have three tasks, task two can’t start until task one is done, and task three can’t start until task two is done.
Asynchronous JavaScript, on the other hand, allows tasks to run independently. This means that task two can start before task one finishes, and task three can start before task two finishes. This enhances the speed and performance of your code.
Also Read: What is Asynchronous JavaScript?
Why Asynchronous JavaScript is Important
Asynchronous JavaScript is crucial for creating fast, responsive web applications. It allows your code to handle multiple operations simultaneously, such as fetching data from a server, updating the user interface, and processing user inputs.
2. Understanding Callbacks
Explanation of Callback Functions
A callback function is a function that is passed as an argument to another function and is executed after some event or task is completed. Callbacks are one of the first methods to manage asynchronous tasks in JavaScript.
Example:
function fetchData(callback) {
setTimeout(() => {
callback("Data fetched!");
}, 2000);
}
function displayData(data) {
console.log(data);
}
fetchData(displayData);
In the above example, fetchData
simulates fetching data from a server and uses setTimeout
to wait 2 seconds. Once the data is “fetched”, it calls the displayData
function to display the data.
Problems with Callback Hell
While callbacks are useful, they can lead to “callback hell” when you have multiple asynchronous operations that depend on each other. This complicates the readability and upkeep of your code.
Example:
fetchData((data) => {
processData(data, (processedData) => {
saveData(processedData, (savedData) => {
console.log(savedData);
});
});
});
This kind of nested structure is difficult to follow and can become a nightmare to debug.
3. Introduction to Promises
What are Promises?
Promises are a simple way to handle asynchronous operations. A promise represents a value that may be accessible immediately, later, or not at all.
Promise States
Promises have three states:
- Pending: The starting condition, neither satisfied nor declined.
- Fulfilled: The operation was completed successfully.
- Rejected: The operation failed.
Creating and Using Promises
Here’s the code to create and use a promise:
let promise = new Promise((resolve, reject) => {
let success = true;
if (success) {
resolve("Operation successful!");
} else {
reject("Operation failed.");
}
});
promise
.then((message) => {
console.log(message);
})
.catch((error) => {
console.error(error);
});
In the above example, if the operation is successful, the promise is fulfilled with the message “Operation successful!” Consequently, it is declined along with the note, “Operation unsuccessful.”
4. Advanced Promise Usage
Promise Chaining
Promise chaining allows you to perform a sequence of asynchronous operations one after another,
fetchData()
.then((data) => processData(data))
.then((processedData) => saveData(processedData))
.then((savedData) => console.log(savedData))
.catch((error) => console.error(error));
Each then
method returns a new promise, allowing you to chain additional asynchronous operations.
Error Handling with Promises
You can handle errors in promises using the catch
method.
Example:
fetchData()
.then((data) => processData(data))
.catch((error) => console.error("Error processing data:", error));
The catch
method will catch any error that occurs in the promise chain.
Using finally
with Promises
The finally
method executes code after the promise has been settled, regardless of whether it was fulfilled or rejected.
fetchData()
.then((data) => processData(data))
.catch((error) => console.error("Error:", error))
.finally(() => console.log("Operation complete."));
5. Async/Await Syntax
Introduction to Async/Await
Async/Await introduces a modern approach to managing tasks that don’t happen at the same time. It enables you to create code that seems and acts as if it were synchronous, even though it’s not.
Converting Promises to Async/Await
Here’s how you can convert promise-based code to async/await:
async function fetchData() {
let response = await fetch("https://api.example.com/data");
let data = await response.json();
return data;
}
fetchData()
.then((data) => console.log(data))
.catch((error) => console.error(error));
The await
keyword stops the function’s operation until the promise is fulfilled.
6. Practical Applications
Real-world Scenarios: Fetching Data from APIs
One of the most common uses of asynchronous JavaScript is fetching data from APIs.
async function getData() {
try {
let response = await fetch("https://api.example.com/data");
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching data:", error);
}
}
getData();
In this example, the getData
function fetches data from an API and logs it to the console.
Example: Building a Mini Project Using Async/Await
Now, let’s build a mini weather app that fetches weather data from an API and displays it.
async function getWeather(city) {
try {
let response = await fetch(`https://api.weather.com/v3/wx/forecast/daily/5day?city=${city}`);
let weather = await response.json();
console.log(`The weather in ${city} is ${weather.description}`);
} catch (error) {
console.error("Error fetching weather data:", error);
}
}
getWeather("New York");
7. Advanced Error Handling Techniques
Handling Errors in Async Functions
You can manage exceptions in asynchronous functions with try/catch blocks.
async function fetchData() {
try {
let response = await fetch("https://api.example.com/data");
if (!response.ok) {
throw new Error("Network response was not ok");
}
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Fetch error:", error);
}
}
fetchData();async function fetchData() {
try {
let response = await fetch("https://api.example.com/data");
if (!response.ok) {
throw new Error("Network response was not ok");
}
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Fetch error:", error);
}
}
fetchData();
Using try/catch with Async/Await
The try/catch block in async functions allows you to handle errors just like in synchronous code.
async function getUserData(userId) {
try {
let response = await fetch(`https://api.example.com/user/${userId}`);
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching user data:", error);
}
}
getUserData(1);
8. Visual Aids and Interactive Elements
Event Loop and Promise State Diagrams
To Understand how asynchronous JavaScript works, it’s helpful to visualize the event loop and promise states.
Event Loop:
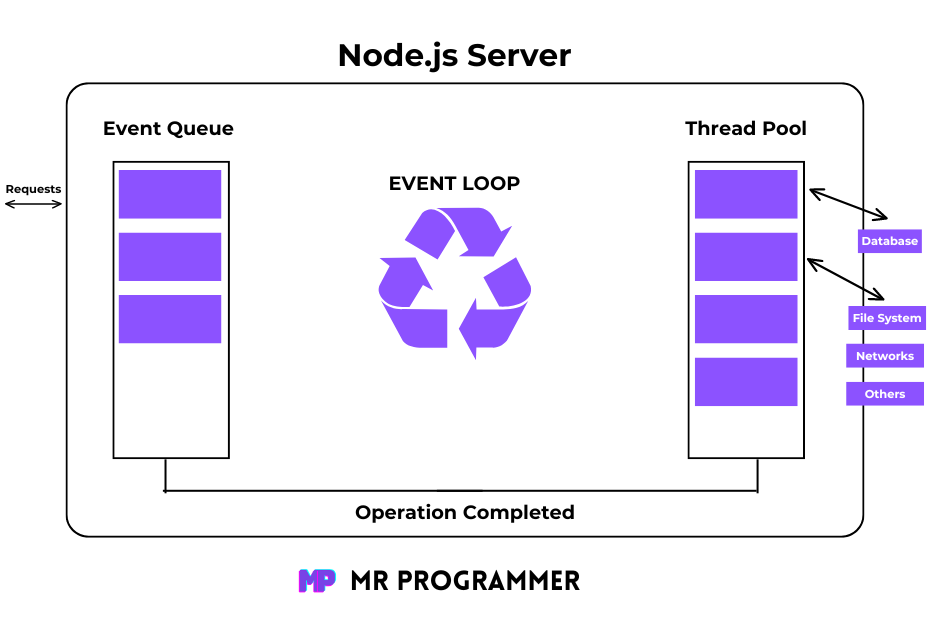
The event loop is a process that constantly checks if the call stack is empty and then adds the next task from the task queue to the stack.
Promise States:
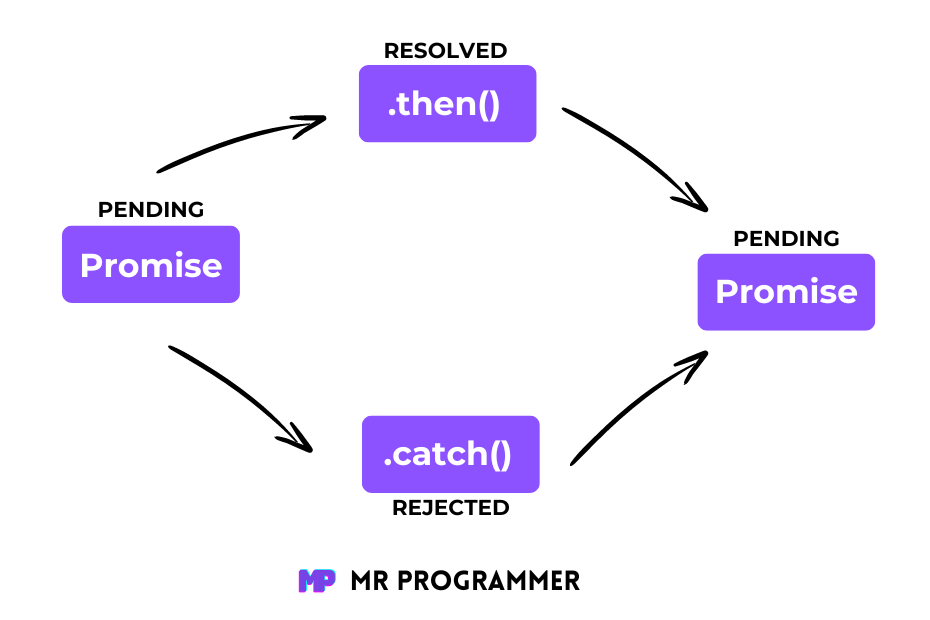
Interactive Coding Challenges and Quizzes
Interactive coding challenges and quizzes can help reinforce your understanding of asynchronous JavaScript. Websites like Codecademy and freeCodeCamp offer great resources for practice.
9. Best Practices and Performance Tips
Avoid Common Pitfalls in Asynchronous Code
- Avoid callback hell: Use promises or async/await to avoid deeply nested callbacks.
- Handle errors properly: Incorporate mechanisms for managing errors in your code that runs asynchronously.
- Don’t block the main thread: Keep your asynchronous code non-blocking to maintain a responsive user interface.
Optimizing Performance in Async Functions
- Batch requests: If you need to make multiple requests, try batching them to reduce the number of round trips.
- Use caching: Cache results of expensive asynchronous operations to avoid redundant processing.
10. Real-World Use Cases
Case Studies of Asynchronous JavaScript in Modern Web Applications
Asynchronous JavaScript is used heavily in modern web applications for tasks like fetching data from APIs, handling user inputs, and updating the user interface.
Example: Asynchronous JavaScript is used in single-page applications (SPAs) to load content dynamically without refreshing the page. This makes SPAs faster and more responsive.
Final Words
Implementing Asynchronous JavaScript Techniques into your coding practices can significantly boost the speed and efficiency of your applications. By understanding and utilizing callbacks, promises, and async/await, you can handle multiple operations simultaneously without slowing down your user interface. These techniques not only make your code faster but also more responsive and user-friendly. Whether you’re fetching data from an API or building complex web applications, mastering these asynchronous methods will help you create smoother and more efficient experiences for your users. So, start applying these techniques today, and watch your JavaScript take off.
Other Related Resources:
- Introduction to Entrepreneurship: Everything You Need to Know Before You Start - June 12, 2025
- What Sets Artificial Intelligence Apart from Humans? - April 18, 2025
- Python Programming: A Comprehensive Guide for Beginners - March 27, 2025